In the modern landscape of web development, RESTful APIs have become indispensable. They enable seamless communication between different software systems, facilitating the creation of scalable and maintainable applications. Java, with its robustness and extensive libraries, and Spring Boot, with its simplified setup and powerful tools, are excellent choices for building RESTful APIs. This article aims to guide you through mastering RESTful APIs using Java and Spring Boot, from understanding the basics to deploying a fully functional API.
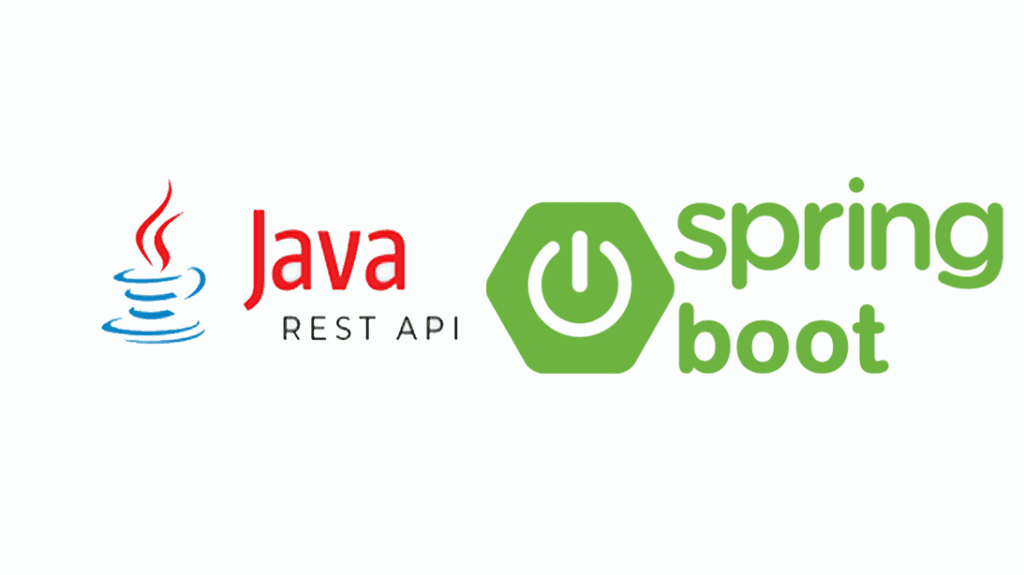
Understanding RESTful APIs
What is REST?
REST, or Representational State Transfer, is an architectural style designed for networked applications. It relies on a stateless, client-server communication protocol, typically HTTP. REST allows for the creation of lightweight, maintainable, and scalable services.
Key Principles of REST
- Statelessness: Each request from a client to a server must contain all the information the server needs to fulfill that request.
- Client-Server Architecture: Separation of concerns between the client (user interface) and the server (data storage).
- Uniform Interface: Standard methods for interacting with resources, such as GET, POST, PUT, and DELETE.
- Resource-Based Interactions: Everything is considered a resource, and each resource is accessible via a unique URL.
HTTP Methods and Status Codes
- GET: Retrieve information from the server.
- POST: Submit data to be processed to the server.
- PUT: Update a resource on the server.
- DELETE: Remove a resource from the server.
- Common Status Codes:
- 200 OK: Request succeeded.
- 404 Not Found: Resource not found.
- 500 Internal Server Error: Server encountered an error.
Why Java and Spring Boot for RESTful APIs?
Java’s Strengths
Java’s platform independence, robustness, and extensive standard libraries make it a strong candidate for building reliable and scalable applications. Its strong typing and object-oriented nature contribute to maintainable code.
Spring Boot’s Advantages
Spring Boot simplifies the setup and configuration of new Spring applications with features like auto-configuration and embedded servers. It provides built-in tools for quickly building RESTful APIs, reducing boilerplate code and accelerating development.
Setting Up Your Development Environment
Installing Java and Spring Boot
To get started, you need to install the Java Development Kit (JDK) and set up Spring Boot. Use Spring Initializr to generate a new Spring Boot project with the necessary dependencies, such as Spring Web.
Creating a Spring Boot Project
Create a new Spring Boot project using Spring Initializr, selecting dependencies like Spring Web. This will generate a basic project structure with essential configurations.
Building a RESTful API with Spring Boot
Defining the API Structure
Plan your API endpoints, resources, and data models. For example, a task management system might have endpoints for tasks, users, and projects.
Creating Controllers
Use the @RestController
annotation to define a controller class and @RequestMapping
to map HTTP requests to handler methods.
@RestController
@RequestMapping("/api/tasks")
public class TaskController {
// Endpoint methods
}
Implementing CRUD Operations
Implement Create, Read, Update, and Delete (CRUD) operations using appropriate HTTP methods.
@PostMapping
public ResponseEntity<Task> createTask(@RequestBody Task task) {
// Create task logic
}
@GetMapping("/{id}")
public ResponseEntity<Task> getTask(@PathVariable Long id) {
// Retrieve task logic
}
Handling Request and Response
Use annotations like @RequestBody
, @PathVariable
, and @RequestParam
to manage input and output data.
@PutMapping("/{id}")
public ResponseEntity<Task> updateTask(@PathVariable Long id, @RequestBody Task task) {
// Update task logic
}
Advanced Features for Mastering RESTful APIs
Data Validation
Use annotations like @Valid
and @NotNull
to validate incoming data and ensure data integrity.
public ResponseEntity<Task> createTask(@Valid @RequestBody Task task) {
// Create task logic
}
Exception Handling
Implement global exception handling with @ControllerAdvice
and @ExceptionHandler
to manage errors gracefully.
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(ResourceNotFoundException.class)
public ResponseEntity<?> handleResourceNotFound(ResourceNotFoundException ex) {
// Handle exception
}
}
Pagination and Sorting
Add pagination and sorting to handle large datasets efficiently, improving performance and user experience.
Security
Secure your API with Spring Security, implementing authentication and authorization mechanisms to protect your resources.
Documentation
Use Swagger or OpenAPI to document your API, making it easier to test and collaborate with other developers.
Testing Your RESTful API
Unit Testing
Write unit tests for controllers and services using JUnit and Mockito to ensure your API functions as expected.
Integration Testing
Test the entire API flow using tools like Postman or automated tests with Spring Boot Test to verify end-to-end functionality.
Deploying Your RESTful API
Packaging the Application
Build a JAR or WAR file using Maven or Gradle to package your application for deployment.
Deploying to a Server
Deploy your API to a cloud platform like AWS or Heroku, or to a local server, making it accessible to users.
Best Practices for RESTful API Development
- Use meaningful endpoint names and follow REST conventions.
- Version your APIs to ensure backward compatibility.
- Optimize performance with caching and efficient database queries.
- Monitor and log API usage for debugging and analytics.
In Short
RESTful APIs are crucial for modern web development, and Java and Spring Boot provide powerful tools for building them efficiently. By following best practices and leveraging advanced features, you can create robust and scalable APIs.
Additional Resources
Top 10 Java Frameworks – For Developer Should Know
Java for Beginners: Key Concepts You Need to Know
Pingback: Java for Big Data: Integration with Hadoop and Spark - WP Pine