Machine learning algorithms, therefore, remain the central idea of artificial intelligence. They make a computer learn from data and decide without explicit instruction; they form the backbone of artificial intelligence. Those powerful tools are changing things around in industries worldwide—from healthcare and finance to marketing and more.
We will explore the world of machine learning algorithms, discovering the various types, how they work, and their multiple applications. We will then provide information about their implementation, making this a complete guide on the subject and one of potential interest.
Introduction to Machine Learning Algorithms
Overview of Machine Learning Algorithms
Machine Learning Algorithms are well-structured rules or procedures that help process patterns through learning. That is, the machine analyzes data and makes some decisions based on knowledge or predicts something. They are an important aspect of modern technology.
Its applications extend from identifying objects in images to understanding human language. Machine learning algorithms are the building blocks for everything that ranges from image and speech recognition to natural language processing or personalized recommendation systems. Today’s data-driven world cannot do without them.
History and Evolution of Machine Learning Algorithms
The development of the machine learning algorithm has its roots in the mid-20th century. Some key milestones marked its early progress. For example, the perceptron laid down the foundations for neural networks during the 1950s. The invention of backpropagation in the 1980s diversified how algorithms learned and improved.
Advances in computational power and the availability of large datasets have propelled their development. These factors have increased the complexity and capabilities of machine learning algorithms to unprecedented levels, making them indispensable for solving modern problems.
Types of Machine Learning Algorithms
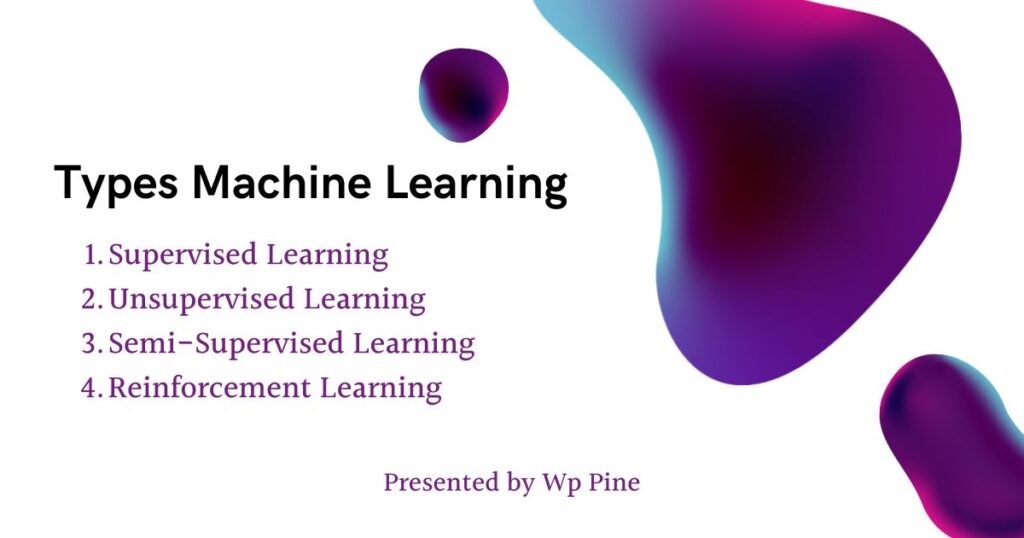
Supervised Learning
Supervised Learning algorithms are trained on labeled data, meaning the algorithm learns from input-output pairs. Common algorithms include:
- Linear Regression: Used for predicting continuous values.
- Logistic Regression: Used for binary classification problems.
- Decision Trees: Tree-like models for decision-making.
- Support Vector Machines (SVM): Describes data by finding the best hyperplane.
- K-Nearest Neighbors (KNN): Classifies data points based on their proximity to other data points.
Unsupervised Learning
Unsupervised Learning algorithms deal with data that has not been categorized or classified and try to discover some kind of clustering or structure inherent in the supplied data.
- K-Means Clustering: Groups data into clusters based on similarity.
- Hierarchical Clustering: Creates a hierarchy of clusters.
- Principal Component Analysis (PCA): Assists in avoiding high data dimensionality.
- Association Rules: Estimates the relation between variables contained in massive datasets.
Semi-Supervised Learning
Semi-supervised learning algorithms rely on a small amount of labeled data with a large amount of unlabeled data. This approach is practical when labeling data is expensive or time-consuming. Common algorithms include:
- Self-Training: Uses its predictions to train further.
- Co-Training: Uses multiple views of the data for training.
- Graph-Based Methods: Uses graph structures to represent data relationships.
Reinforcement Learning
Reinforcement Learning algorithms train agents to make sequences of decisions by rewarding them for good choices and penalizing them for bad ones. Common algorithms include:
- Q-Learning: Uses a value-based approach for action selection.
- SARSA: Similar to Q-Learning but updates the action-value function based on the action taken.
- Deep Q-Networks (DQN): Combines Q-Learning with deep learning.
- Policy Gradient Methods: Improve policy instead of the value function, which is more computation-intensive.
Mostly Used Machine Learning Algorithms
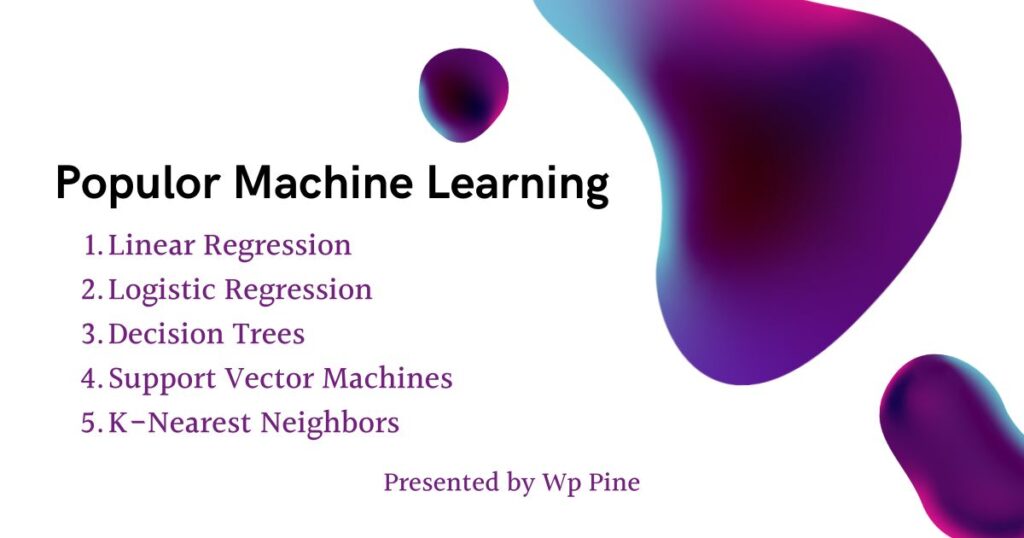
Linear Regression
Linear regression is considered the least complex but most widely used machine learning algorithm. Its main application is predicting a dependent continuous variable based on one or more independent variables.
This algorithm’s model represents a linear equation describing the relationship between variables. It seeks the best-fit line with the minimum error for actual and predicted values. Due to its simplicity and effectiveness, linear regression has popular applications in sales forecasting, risk assessment, and trend analysis.
The formula for a simple linear regression is:
[ y = beta_0 + beta_1 x + epsilon ]
where ( y ) is the dependent variable, ( x ) is the independent variable, ( beta_0 ) and ( beta_1 ) are the coefficients, and ( epsilon ) is the error term.
Use Cases: Linear Regression is used in finance to predict stock prices, in marketing to forecast sales, and in various other fields to analyze trends.
Implementation Example:
from sklearn.linear_model import LinearRegression
import numpy as np
# Sample data
X = np.array([[1], [2], [3], [4], [5]])
y = np.array([1, 3, 2, 5, 4])
# Create and train the model
model = LinearRegression()
model.fit(X, y)
# Make predictions
predictions = model.predict(np.array([[6]]))
print(predictions)
Logistic Regression
Logistic Regression is one of the base algorithms constructed solely for binary classification. It gives the probability that an event happens where the outcome belongs to one of the classes. Usually, one class represents the event while the other represents its absence.
This algorithm uses the logistic function, or the sigmoid function, to map predicted values into probabilities. If it generates probabilities, it will determine how likely it is for an observation to belong to a specific class. Its popularity is attributed to logistic regression’s simplicity and interpretability, which makes it very suitable for spam detection, disease diagnosis, and customer churn prediction.
Use Cases: Logistic Regression is widely used for credit scoring, medical diagnosis, and spam detection.
Implementation Example:
from sklearn.linear_model import LogisticRegression
import numpy as np
# Sample data
X = np.array([[1], [2], [3], [4], [5]])
y = np.array([0, 0, 0, 1, 1])
# Create and train the model
model = LogisticRegression()
model.fit(X, y)
# Make predictions
predictions = model.predict(np.array([[6]]))
print(predictions)
Decision Trees
Decision Trees are versatile algorithms in machine learning used for classification and regression tasks. The data set is split according to the input values of features. It is a tree-like structure where the node points are decision points, and the branches will output.
It features the choice that best separates the data at each step, allowing clear and logical decisions. Decision Trees are valued for their simplicity, interpretability, and effectiveness in handling complex datasets. They are broadly applied in customer segmentation, credit scoring, and medical diagnosis.
Use Cases: Decision Trees are used in customer segmentation, risk analysis, and medical diagnosis.
Implementation Example:
from sklearn.tree import DecisionTreeClassifier
import numpy as np
# Sample data
X = np.array([[1], [2], [3], [4], [5]])
y = np.array([0, 0, 0, 1, 1])
# Create and train the model
model = DecisionTreeClassifier()
model.fit(X, y)
# Make predictions
predictions = model.predict(np.array([[6]]))
print(predictions)
Support Vector Machines (SVM)
It’s a very powerful classification algorithm called the Support Vector Machine. The basic idea of a support vector machine is to find an optimal hyperplane that would segregate examples into different classes such that the margins, or distances, between classes are maximized for proper discrimination.
It performs excellently with high-dimensional data, making SVMs extremely effective for complex problems. They have wide applications in fields such as text categorization, where documents fall into various topics, and image recognition, in which objects are put together with precision. Even with the kernel functions making it possible to manipulate non-linear boundaries, their applicability is greatly extended.
Use Cases: SVMs are used in text and hypertext categorization, image classification, and bioinformatics.
Implementation Example:
from sklearn.svm import SVC
import numpy as np
# Sample data
X = np.array([[1], [2], [3], [4], [5]])
y = np.array([0, 0, 0, 1, 1])
# Create and train the model
model = SVC()
model.fit(X, y)
# Make predictions
predictions = model.predict(np.array([[6]]))
print(predictions)
K-Nearest Neighbors (KNN)
KNN is one of those very simple, non-parametric classification and regression algorithms; it classifies the data points based on the majority class among the k-nearest neighbors in the database.
An algorithm identifies the ‘k’ closest points to it, which usually rely on distance metrics such as Euclidean distance when a new data point is presented. The class or assigned value to the new point relies on its neighbors’ most prevailing class or average value. KNN is valued for its simplicity and ease of implementation; this is what makes it so popular among other applications that include recommendation systems and pattern recognition.
Use Cases: KNN is used in recommendation systems, image recognition, and video recognition.
Implementation Example:
from sklearn.neighbors import KNeighborsClassifier
import numpy as np
# Sample data
X = np.array([[1], [2], [3], [4], [5]])
y = np.array([0, 0, 0, 1, 1])
# Create and train the model
model = KNeighborsClassifier(n_neighbors=3)
model.fit(X, y)
# Make predictions
predictions = model.predict(np.array([[6]]))
print(predictions)
K-Means Clustering
K-means clustering is an unsupervised learning algorithm that groups data into K-distinct clusters. Each point is assigned to the cluster whose mean or centroid is closest.
The initialization process starts by generating k centroids; the clusters are iteratively improved by sending each data point to the nearest centroid and updating the centroids by the new members in each cluster. This is repeated until the clusters stabilize so each data point is in the best possible group. K-means is widely used in customer segmentation, market research, and image compression because of its efficiency and simplicity.
Use Cases: K-means clustering is used in customer segmentation, market segmentation, and image compression.
Implementation Example:
from sklearn.cluster import KMeans
import numpy as np
# Sample data
X = np.array([[1], [2], [3], [4], [5]])
# Create and train the model
model = KMeans(n_clusters=2)
model.fit(X)
# Get cluster centers and labels
centers = model.cluster_centers_
labels = model.labels_
print(centers, labels)
Advanced Machine Learning Algorithms
Neural Networks
Neural networks are algorithms inspired by the structure and operation of the human brain. They were designed for pattern recognition but can also be used for prediction. Therefore, their real application is primarily in complex tasks like image recognition, speech processing, and natural language understanding.
A neural network consists of several layers of connected nodes, or “neurons,” that process and forward information. Major types of neural networks include feed-forward networks, which pass information only in one direction; convolutional neural networks, which are widely adopted for image recognition; and recurrent neural networks, which are particularly suited for tasks involving sequential data, such as speech, text, etc.
These networks revolutionized everything other than computer visions and self-driving cars to voice assistants, and they became essentials in AI development.
Gradient Boosting Machines (GBM)
A Gradient Boosting Machine is an ensemble learning technique wherein models are built sequentially. The process trains each new model to correct the previous model’s errors and improve it incrementally.
The iterative approach reduces GBM’s bias and variance, making it very useful in regression and classification tasks. GBM captures complex patterns in data by capitalizing on the strengths of several models. Hence, high-performance predictive modeling in various domains, such as finance, healthcare, and marketing, is widely used.
Random Forests
Random Forest is an ensemble learning method that combines classification and regression tree techniques. In this method, the learning algorithm creates multiple decision trees during training and then merges their predictions to improve accuracy.
Results from many decision trees are aggregated to reduce overfitting risk in individual decision trees. Hence, Random Forest is a robust algorithm that can handle large datasets with high dimensionality. It is widely used in tasks such as risk analysis, fraud detection, and predictive modeling, which offer very high accuracy and versatility.
Evaluation of Machine Learning Algorithms
Model Performance Metrics
Several key performance metrics can be used when evaluating machine learning models. These mainly include accuracy, precision, recall, F1-score, and ROC-AUC, which help understand how a model works differently.
- Accuracy is about the overall correctness of the model.
- Precision is talking about how many predicted positive instances are indeed positive.
- Recall is how many actual positive instances were correctly found.
- F1-score balances both precision and recall against one another to provide a single measure for evaluating model performance across imbalanced datasets.
- ROC-AUC: This evaluates the model’s ability to distinguish between positive and negative classes at different thresholds.
Another critical aspect is the cross-validation of the model’s performance. This means training your model on multiple subsets or folds and splitting your dataset into subsets. This helps avoid overfitting so that your model generalizes well to unseen data and gives a more accurate evaluation of your actual performance.
Model Selection and Hyperparameter Tuning
Techniques such as grid search, random search, and Bayesian optimization are traditionally used to tune the best model and fine-tune the appropriate hyperparameters. This helps control bias and variance, which improves model performance.
- Grid Search exhaustively searches across a predefined set of hyperparameters, evaluating all possible combinations to arrive at the optimal configuration.
- Random Search randomly draws hyperparameter combinations, which, for large hyperparameter spaces, actually proves more efficient.
- Bayesian Optimization uses probabilistic models to predict the best-performing hyperparameters and explores the space more intelligently and efficiently than grid or random search.
These techniques had the potential to ensure that such a model is properly tuned to minimize error and enhance generalization to unseen data.
Challenges and Considerations
Overfitting and Underfitting
Overfitting describes the scenario when a model does exceptionally well with the training data but fails to generalize to new data – specifically unseen data. The model has memorized the training data, capturing noise and outliers rather than learning the patterns underlying the task.
On the other hand, underfitting occurs because the chosen model is oversimplified, failing to capture the complex data. In most cases, this results in poor learning on both training and test data.
Techniques commonly used to deal with these problems include regularization and cross-validation. Regularization adds a penalty term to the model so that it doesn’t grow too big and begin to overfit. Cross-validation builds on this by ensuring that the model does several subsets of the data to obtain a more reliable estimate of generalizability.
These methods aim to get an optimally balanced model that does well in training and new data.
Scalability and Efficiency
Due to memory and computational constraints, handling large data sets could pose a significant challenge. Techniques such as parallel processing, distributed computing, and efficient algorithms are important for ensuring scalability and optimizing performance in processing and analyzing big data.
- Parallel Processing allows multiple tasks to be executed parallelly, leveraging the power of many processors to speed up computation.
- Distributed computing involves splitting data into parts and processing it on different machines. This enables a user to process big datasets that cannot be handled by one machine.
- These algorithms have been designed to process data with minimal possible resource usage, allowing them to handle large datasets without overloading the system.
By applying these methods, businesses and researchers can process large volumes of information more effectively, making room for timely insights and decision-making.
Interpretability and Explicable
Understanding how the model makes its decisions is very important, especially in high-stakes applications involving healthcare, finance, and legal systems. Techniques like LIME (Local Interpretable Model-agnostic Explanations) and SHAP (Shapley Additive) can be valuable for improving model transparency and obtaining trustworthy results.
- LIME can be considered a simpler, interpretable model that locally approximates the complex model for any prediction. Thus, it enables users to understand what features contribute most to any specific prediction.
- SHAP is a game-theoretic method that provides a unified measure of feature importance. With every feature assigned an importance value for each prediction, it guarantees consistent and accurate explanations of the model’s behavior.
Both LIME and SHAP enhance model interpretability, thereby providing the practitioner with more insight into how the model reached its decision, making the results understandable and justifiable.
Tools and Libraries
The most widely applied libraries for machine learning algorithms implementation come with strong tools for building and deploying models. Scikit-learn, TensorFlow, Keras, and PyTorch are the most popular.
- Scikit-learn is a powerful and intuitive library for classical machine learning, which can be used for classification, regression, and clustering. Those algorithms are supplemented with preprocessing and evaluation tools.
- TensorFlow is open-source software primarily dedicated to deep learning applications. It is also highly scalable and can support some very complex neural network models; thus, it could be used in both research and production environments.
- Keras is a high-level API for neural networks that simplifies its implementation and usage. It’s usually used with TensorFlow, which makes building deep learning models much easier.
- The other deep learning framework is PyTorch, with dynamic computational graphs. This framework quickly gained popularity among researchers due to its ease of experimentation and debugging.
This will provide powered entirely ecosystems for developing, training, and deploying machine learning models, enabling innovative developers and data scientists to create solutions within any domain.
Key Outcomes
Machine learning algorithms have played a crucial role in creating intelligent systems designed to analyze data, learn from it, and act accordingly. The current guide covered some of the various algorithms by explaining their specific applications and usage cases. It also showed the main methods for evaluating a model’s performance, including accuracy, precision, recall, and common challenges when working with machine learning models.
Each algorithm’s strengths, limitations, and appropriate use cases must be clearly understood for their fullest realization in real-world applications.
Further Reading and Resources
To better understand these theories, “Pattern Recognition and Machine Learning” by Christopher Bishop is an all-rounded book for newbies and advanced learners. Some online courses, such as Coursera’s “Machine Learning” by Andrew Ng, can also be taken for practical experiences and a deeper understanding of major concepts and techniques applied in the field.
These rich resources provide excellent insights and practical applications. They are perfect for anyone keen on progressing in machine learning skills.
For more information on machine learning algorithms, visit Scikit-learn Documentation & TensorFlow Tutorials & PyTroch.
- Learn The Power of Reinforcement Learning by Abdul Moeez
- Complete Guide to Evaluate Machine Learning Model by Abdul Moeez
- Machine Learning Vs Meta Learning Explained by Abdul Moeez